π New: Group analytics is getting a makeover! Sign up for the B2B analytics feature preview to get a sneak peek. Let us know what you think in-app.
Groups aggregate events based on entities, such as organizations or companies. They are especially useful for B2B customers and enable you to deploy feature flags, analyze insights, and run experiments at a group-level, as opposed to a user-level.
To clarify what we mean, let's look at a few examples:
For B2B SaaS apps, you can create a company group type. This enables you to aggregate events at a company-level, and calculate metrics such as
number of daily active companies
,company churn rate
, orhow many companies have adopted a new feature
.For a communication app like Slack, you can create a channel group type. This enables you to measure metrics like
average number of messages per channel
,number of monthly active channels
, ortotal number of channel participants
.For collaborative, project-based apps like Notion, Jira, or Figma, you can create a project group type. This enables you to calculate metrics like
project pageviews
,users per project
, andproject engagement
.
Use cases
Building on the examples above, here are a few things you can do with groups:
- Track how companies progress through your B2B product's activation steps. For example, you can create a funnel to see:
- How many companies signed up this month.
- What percentage completed the onboarding flow.
- What percentage of new companies interacted with specific features at least once.
- If you're building a Slack-like app, you can measure retention of new features by specific channels. For example:
- Which channels consistently use the video calling feature.
- How many channels remain active month over month.
- Compare feature adoption rates between different types of channels (e.g., team channels vs project channels).
- For collaborative, project-based apps like Jira, you can target feature flags at a project-level. For example, let's say you've refactored your codebase. You can target the new codebase to only a few projects and measure the impact on performance and errors metrics. Once you've gathered feedback, you can expand to the rest of your projects.
Here's an overview to how groups can be applied across PostHog:
Product | Functionality | Example |
---|---|---|
Product analytics | Aggregate trends, funnels, retention, and stickiness by group. | Create a funnel to track how many companies completed your onboarding flow. |
Feature flags | Configure release conditions based on groups. | Ensure all users of a given company receive the same feature flag variant. |
Experiments | Evaluate experiment results based on group aggregations. | Run an A/B test to improve activation rate for new companies. |
Data warehouse | Join tables or enrich queries with groups data. | Write a custom SQL query that calculates product usage based across different company sizes. |
The difference between groups and cohorts
Groups are often confused with cohorts, but they each serve different purposes:
- Groups aggregate events, and do not necessarily have to be connected to a user.
- Cohorts represent a specific set of users β e.g., a list of users that all belong to the same company.
If your only goal is to analyze a list of users with something in common, we recommend cohorts instead of groups.
Groups require additional code in your app to set up, while cohorts are created in PostHog and don't require additional code. This makes cohorts easier to use and quicker to get started.
How to create groups
Groups must be created before events can be associated with them.
// Call posthog.group() to create a group before capturing events.// It sends a `$groupidentify` event to create or update the group.// It will also create the group type if it doesn't exist. In the// web SDK, it also associates all subsequent events in the session// with the group.posthog.group('company', 'company_id_in_your_db');// This event will be associated with the company above.posthog.capture('user_signed_up');// If the group type is already created, you can also manually add// the `$groups` property to any event you want to associate with// the group.posthog.capture('user_signed_up', {'$groups': {'company': 'company_id_in_your_db'}});
In the above example, we create a group type company
. Then, for each company, we set the group key
as the unique identifier for that specific company. This can be anything that helps you identify it, such as ID or domain.
We now have one company
-type group with a key company_id_in_your_db
. When we send the event user_signed_up
, it will be attached to this newly created group.
Tips:
- When specifying the group type, use the singular version for clarity (
company
instead ofcompanies
).- We advise against using the name of the company (or any other group) as the key, because that's rarely guaranteed to be unique, and thus can affect the quality of analytics data. Use a unique string, like an ID.
Group type limit: There's a hard limit of 5 group types within PostHog, although within each group type you can have an unlimited number of groups.
How to set group properties
In the same way that every person can have properties associated with them, every group can have properties associated with it.
Continuing with the previous example of using company
as our group type, we'll add company_name
, date_joined
, and subscription
as additional properties.
Note: You must include at least one group property for a group to be visible in the People and groups tab.
// Option 1 (recommended): Set properties in posthog.group()// This has the side-effect that all subsequent events in the session are associated to the groupposthog.group('company', 'company_id_in_your_db', {name: 'PostHog',subscription: "subscription",date_joined: '2020-01-23'});// Option 2:// Set properties in posthog.capture()// This method doesn't have the side-effect of associating all future events to the group.posthog.capture('$groupidentify', {'$group_type': 'company','$group_key': 'company_id_in_your_db','$group_set': {name: 'PostHog',subscription: "subscription",date_joined: '2020-01-23'}});
Properties on groups behave in the same way as properties on persons. They can also be used within experiments and feature flags to rollout features to specific groups.
Note: The PostHog UI identifies a group using the
name
property. If thename
property is not found, it falls back to the group key.
How to capture group events
How you capture events with groups depends whether you're using the JavaScript Web SDK or not.
If you're using the JavaScript Web SDK (or snippet), you can call
posthog.group()
and all of that session's events will be associated with that group.If you're using any other SDK (or the API), you need to pass the group information in the
groups
parameter (or$groups
property) for every event you capture.
Below are code examples of how to do it in our various SDKs.
// Call posthog.group() to create a group before capturing events.// It sends a `$groupidentify` event to create or update the group.// It will also create the group type if it doesn't exist. In the// web SDK, it also associates all subsequent events in the session// with the group.posthog.group('company', 'company_id_in_your_db');// This event will be associated with the company above.posthog.capture('user_signed_up');// If the group type is already created, you can also manually add// the `$groups` property to any event you want to associate with// the group.posthog.capture('user_signed_up', {'$groups': {'company': 'company_id_in_your_db'}});
Want to learn more about group analytics implementation differences? Check out our guide on frontend vs backend group analytics implementations.
Events must be identified to be associated with a group. If the $process_person_profile
event property ends up being set to false
, the event will not be associated with the group.
It is NOT possible to assign a single event to two groups of the same type. However, it is possible to assign an event to multiple groups as long as the groups are of different types.
// β Not possibleposthog.group('company', 'company_id_in_your_db');posthog.group('company', 'another_company_id_in_your_db');posthog.capture('user_signed_up')// β Allowedposthog.group('company', 'company_id_in_your_db');posthog.group('channel', 'channel_id_in_your_db');posthog.capture('user_signed_up')
Advanced (server-side only): Capturing group events without a user
If you want to capture group events but don't want to associate them with a specific user, we recommend using a single static string as the distinct ID to capture these events. This can be anything you want, as long as it's the same for every group event:
posthog.capture({event: 'group_event_name',distinctId: 'static_string_for_group_events',groups: { company: 'company_id_in_your_db' }})
Using groups in PostHog
Now that we have created our first group type, we can take a look at how to use groups within PostHog.
Viewing groups and their properties
To view groups and their properties, head to the People and groups tab on the navigation bar.
From here, you can select the group type you are interested in and view the groups and properties (by clicking the chevrons on the left). The properties shown here can be customized by clicking Configure columns.
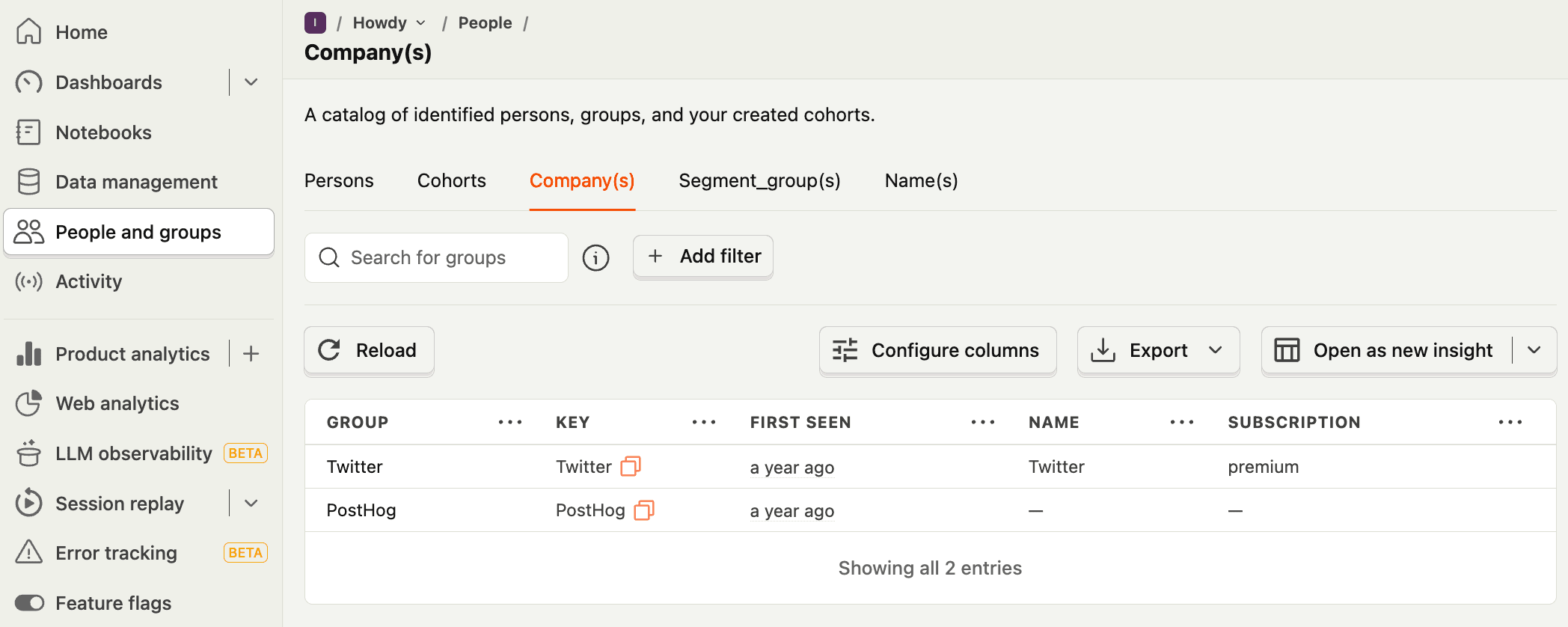
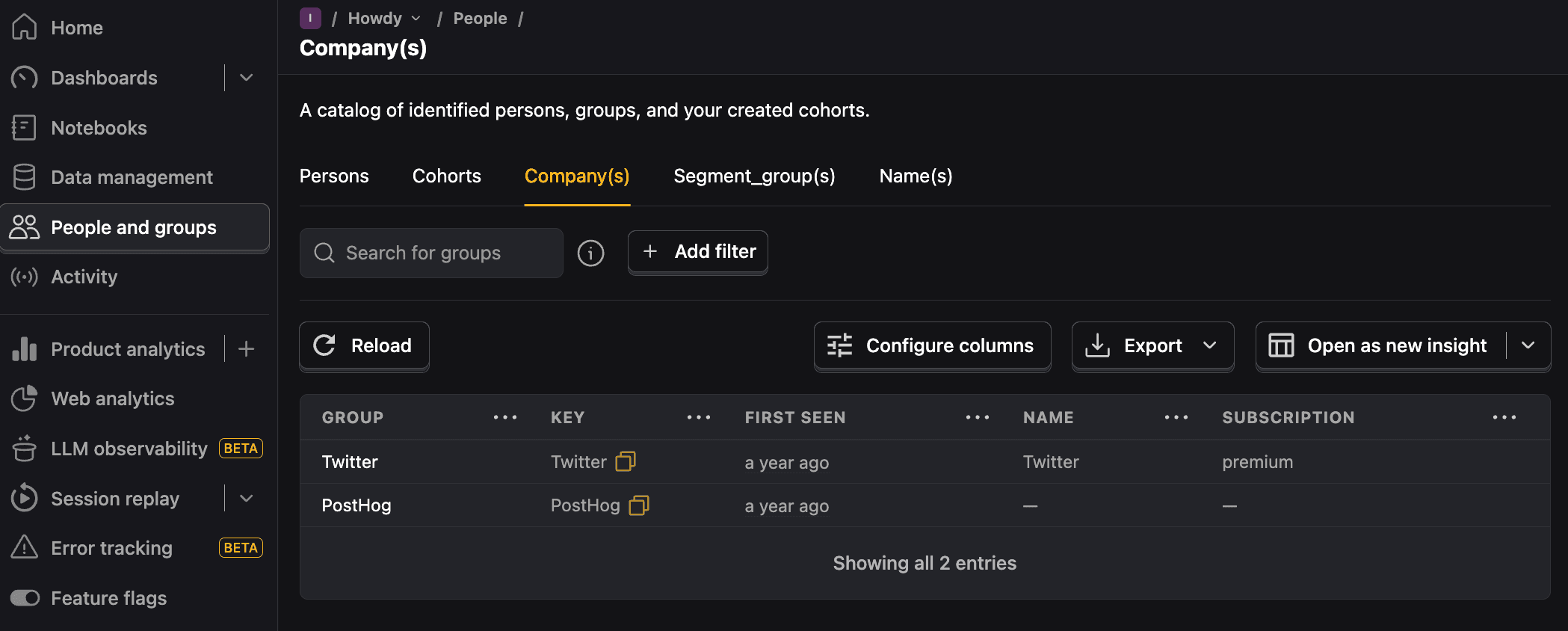
Clicking one of groups brings you to the group details page. Here, you can generate, view, and customize a dashboard for the group. Beyond this, you can see events, recordings, related people, and more. On the properties tab, you can add, update, and delete group properties.
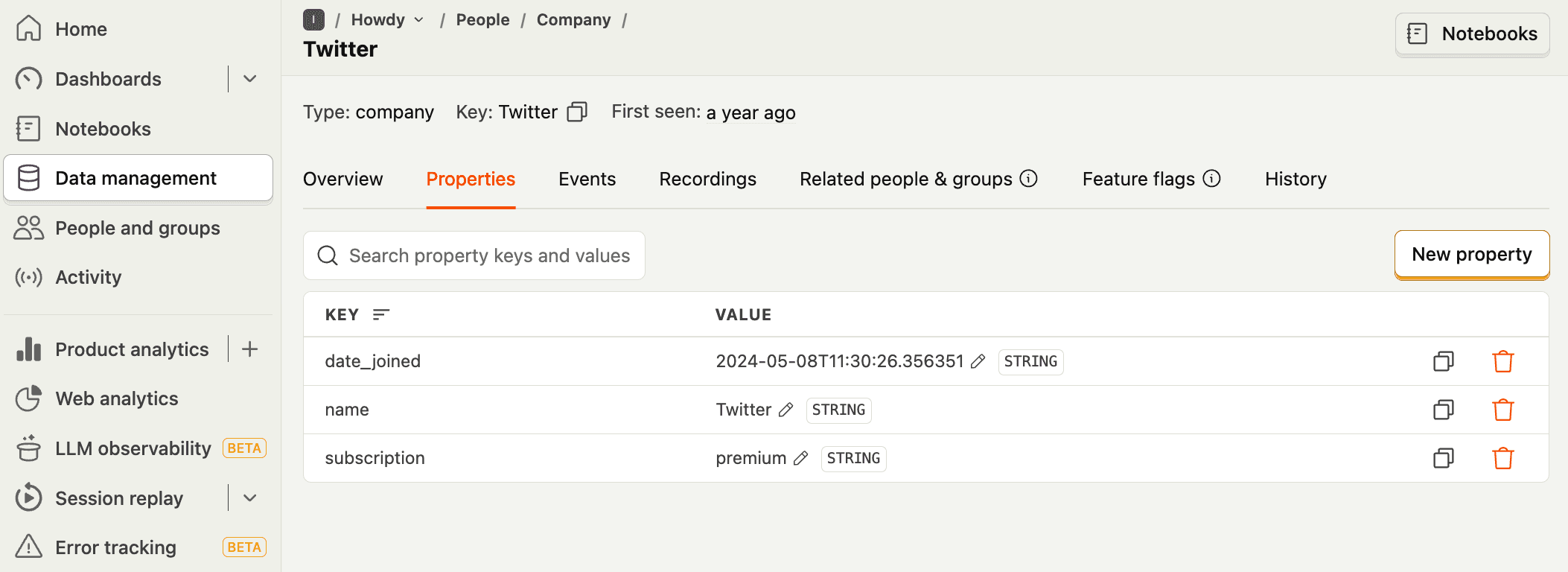
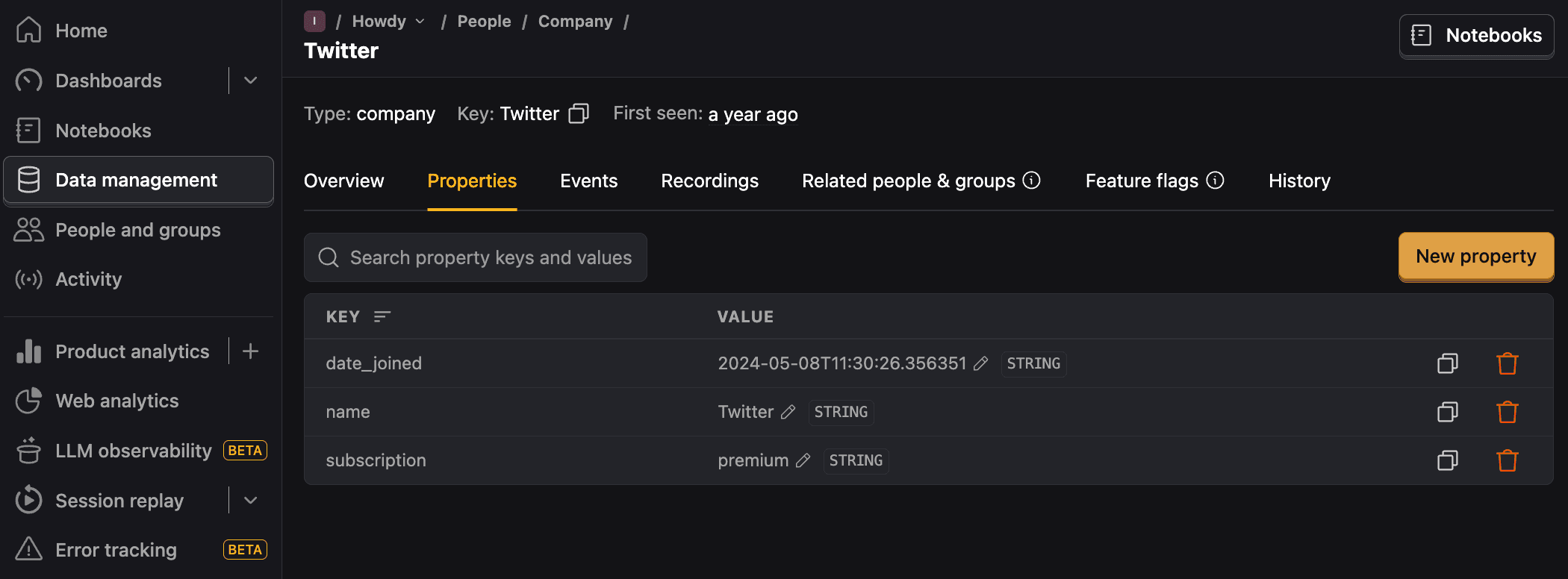
How to view group insights
You can use groups in insights to view aggregated events based on group type.
For example, let's say that we wanted to see a graph showing how many different organizations have signed up recently:
To do this, expand the menu next to the event you've chosen. This lists of all the group types available. Next, select the option for "Unique" with your group type name, such as "Unique organizations." This shows a graph with the total number of groups (organizations) that have signed up (as opposed to individual users).
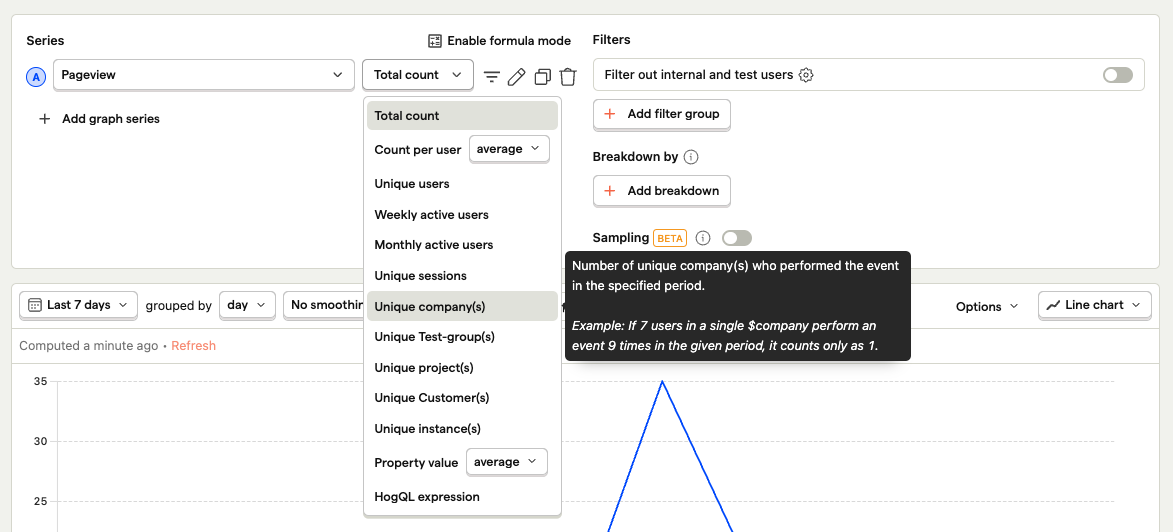
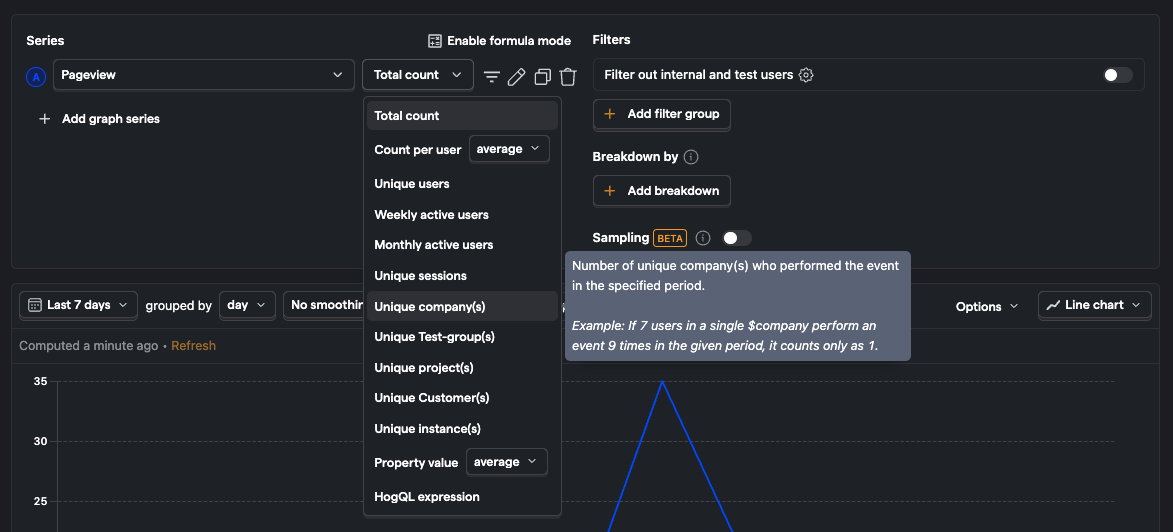
Using groups with funnels
Another place where group analytics can be used is within funnels.
For example, you may want to understand how organizations move from their first visit to eventually signing up. You can do this by setting the "Aggregating by" field to "Unique organizations."
This shows how many organizations have made it through, as well as the percentage of organizations that dropped off. It's also possible to see exactly which specific groups dropped off at each step.
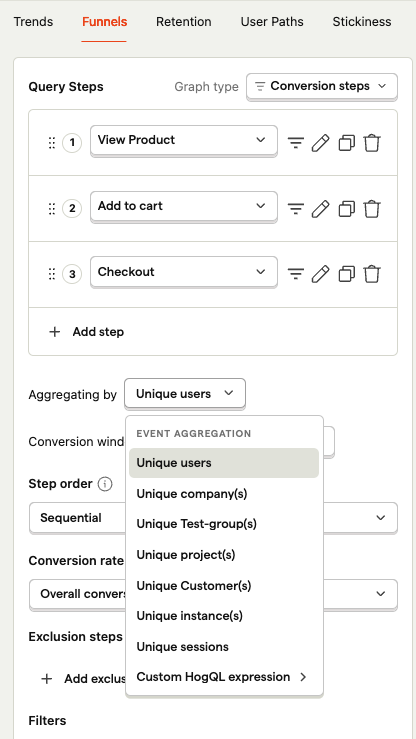
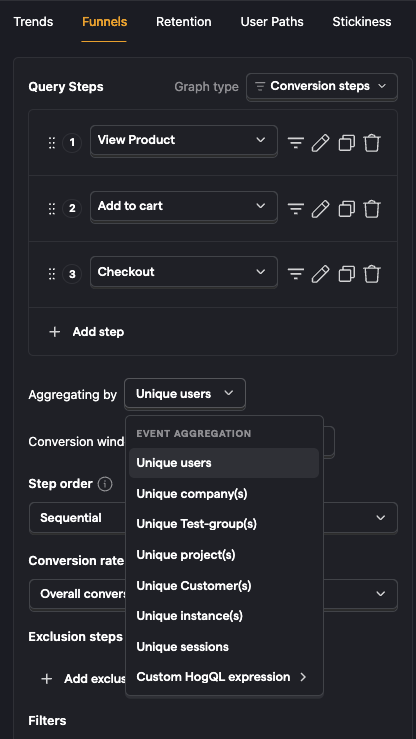
Using groups with feature flags
Groups in feature flags enable you to rollout a feature by group type (like company
), instead of users.
To do this, create a feature flag as you normally would, and select the group type you wish to "Match by", using the drop down in the "Release conditions" section:
You also need to update your event tracking code for the feature flag to determine the groups of the current user.
// Make sure you have called posthog.group() earlier in that sessionif (posthog.isFeatureEnabled('new-groups-feature')) {// Do something}
Renaming group types
You can change how group types are displayed in the insights interface and throughout PostHog by in project settings.
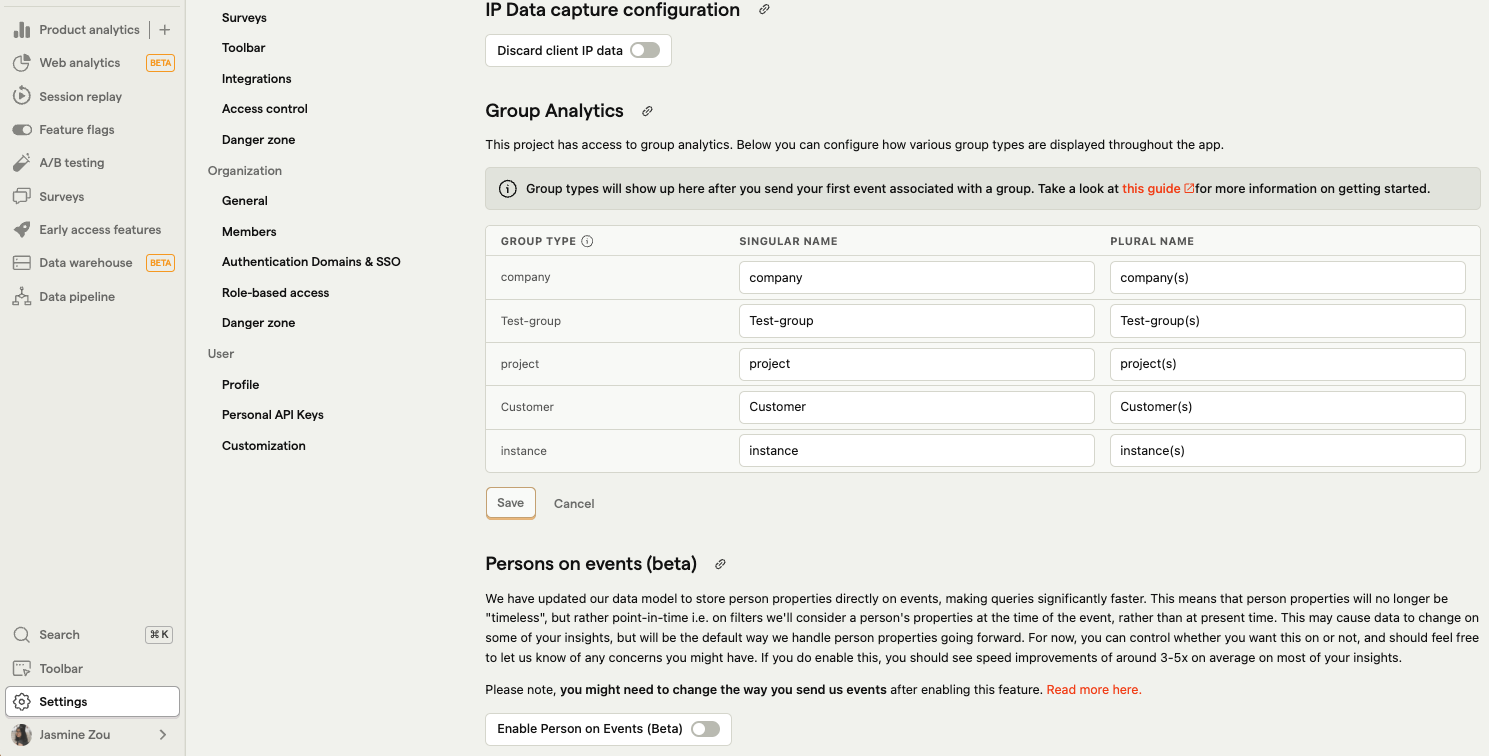
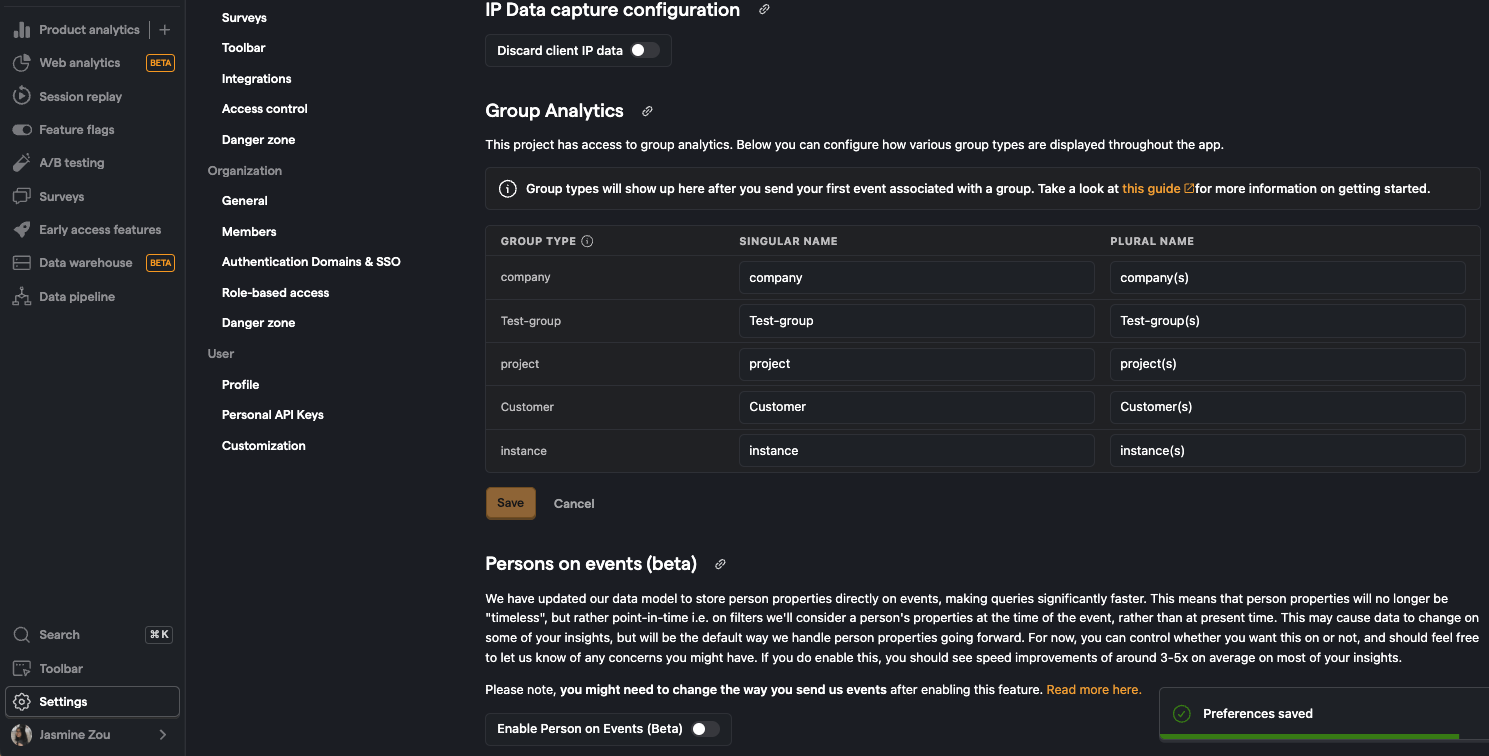
Limitations
- A maximum of 5 group types can be created per project.
- Multiple groups of the same type cannot assigned to a single event (e.g., Company A & Company B).
- Groups are not currently supported for the following insights:
- Lifecycle - Expected soon.
- User paths - These only support user level analytics.
- Only groups with known properties are shown under People and groups .
- Currently there is no functionality within the app to delete groups. If you need to delete a group for privacy or security reasons, please use the support modal.