Note: Early access management is only available in the JavaScript web SDK.
Early access feature management enables your users to opt in (and out) of betas and other in-progress features.
This is useful if you want to:
- Run a beta program without building a custom solution
- Provide access to features that are not yet ready for general release
- Allow users to control their own product experience
How it works
Early access features can be created and edited from the Early Access Management tab in PostHog.
Features are powered by feature flags. Flags are automatically created when a feature is created (based on the name of the feature), or you can link an existing feature flag to a feature. Each feature can also contain a description and documentation link to help users understand what the feature is for.
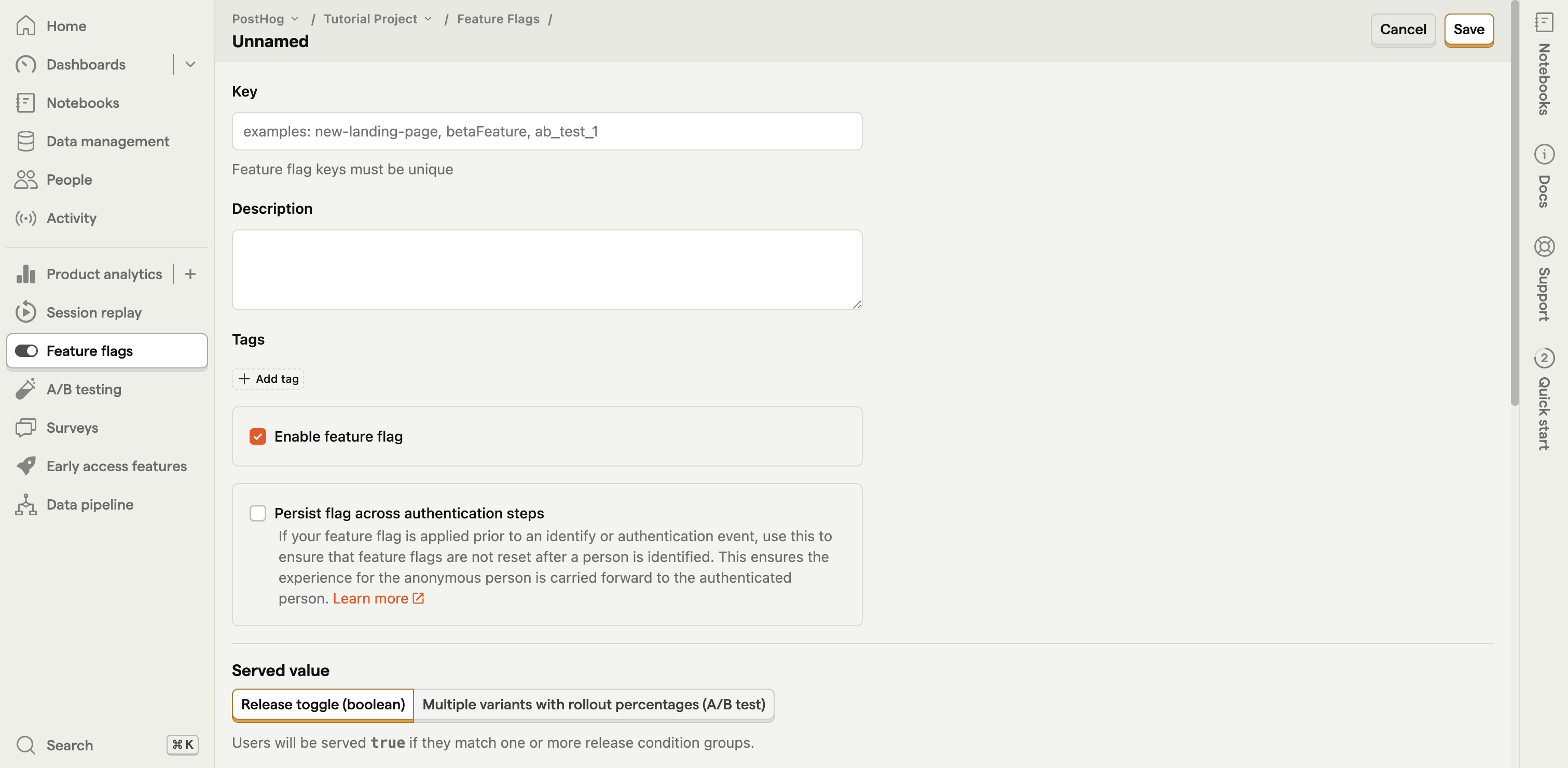
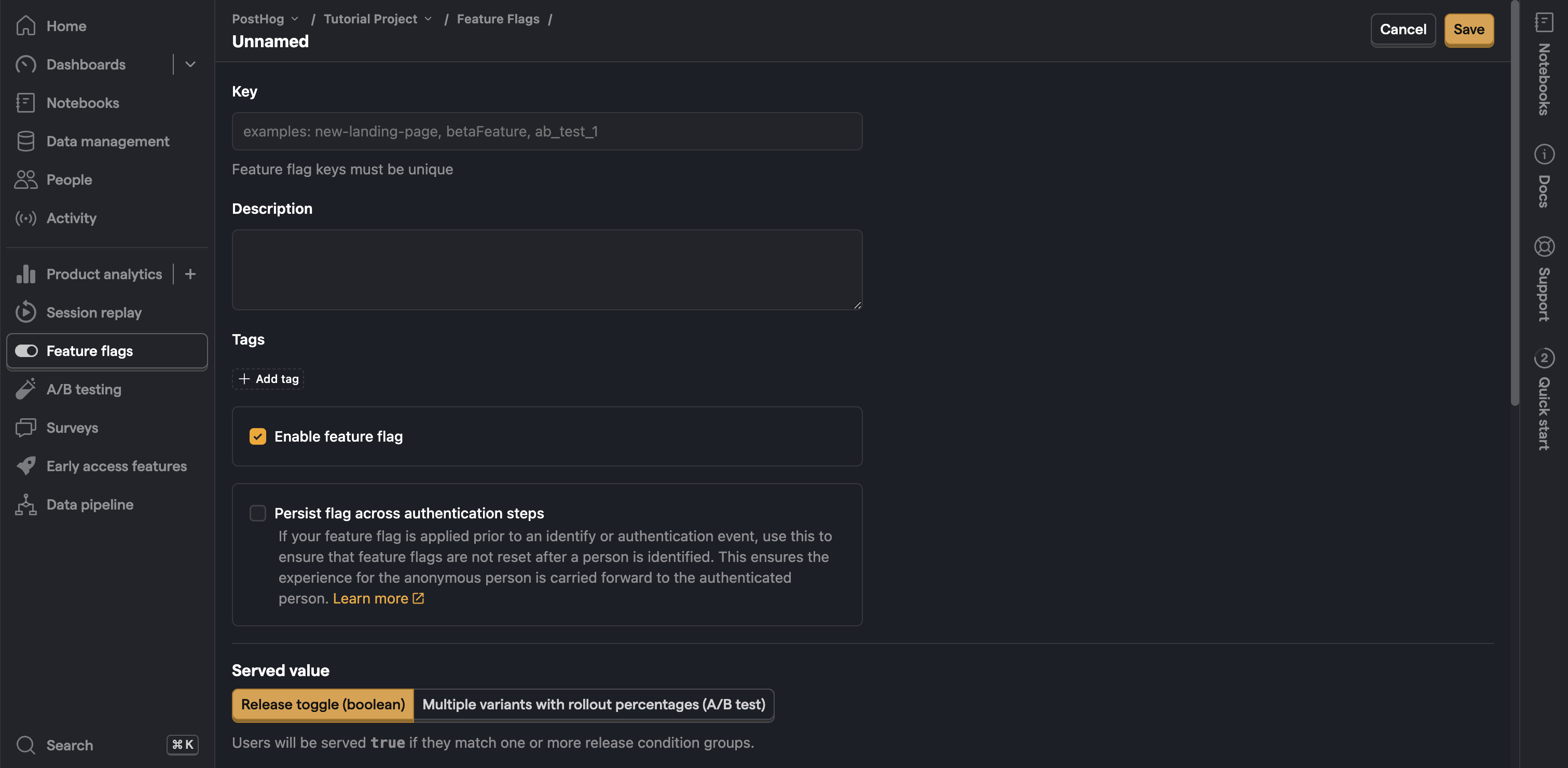
Once created, you can opt users in or out manually on the feature detail screen by searching for them in PostHog. You can also implement a public opt-in flow which we detail below.
Important: Early access feature opt-in is an overriding condition. This means that a user opt-in or opt-out overrides any existing release condition on the feature flag. Only when a user has not explicitly selected to opt in or out will the release conditions on the feature flag be used to determine feature flag availability.
Creating a public opt-in
You can find a full walkthrough of building an app with early access feature management in our How to set up a public beta program using early access management tutorial.
Option 1: Site app
We've prebuilt a site app that adds an early access feature modal to your site.
To use it:
Ensure you set
opt_in_site_apps: true
in your PostHog initialization configuration.Search for Early Access Features App in the Browse Apps tab.
Enable it by clicking the blue gear, setting an HTML attribute selector like
data-attr
,#id
, or.class
(or enabling "Show features button on the page"), enabling the toggle and pressing save.
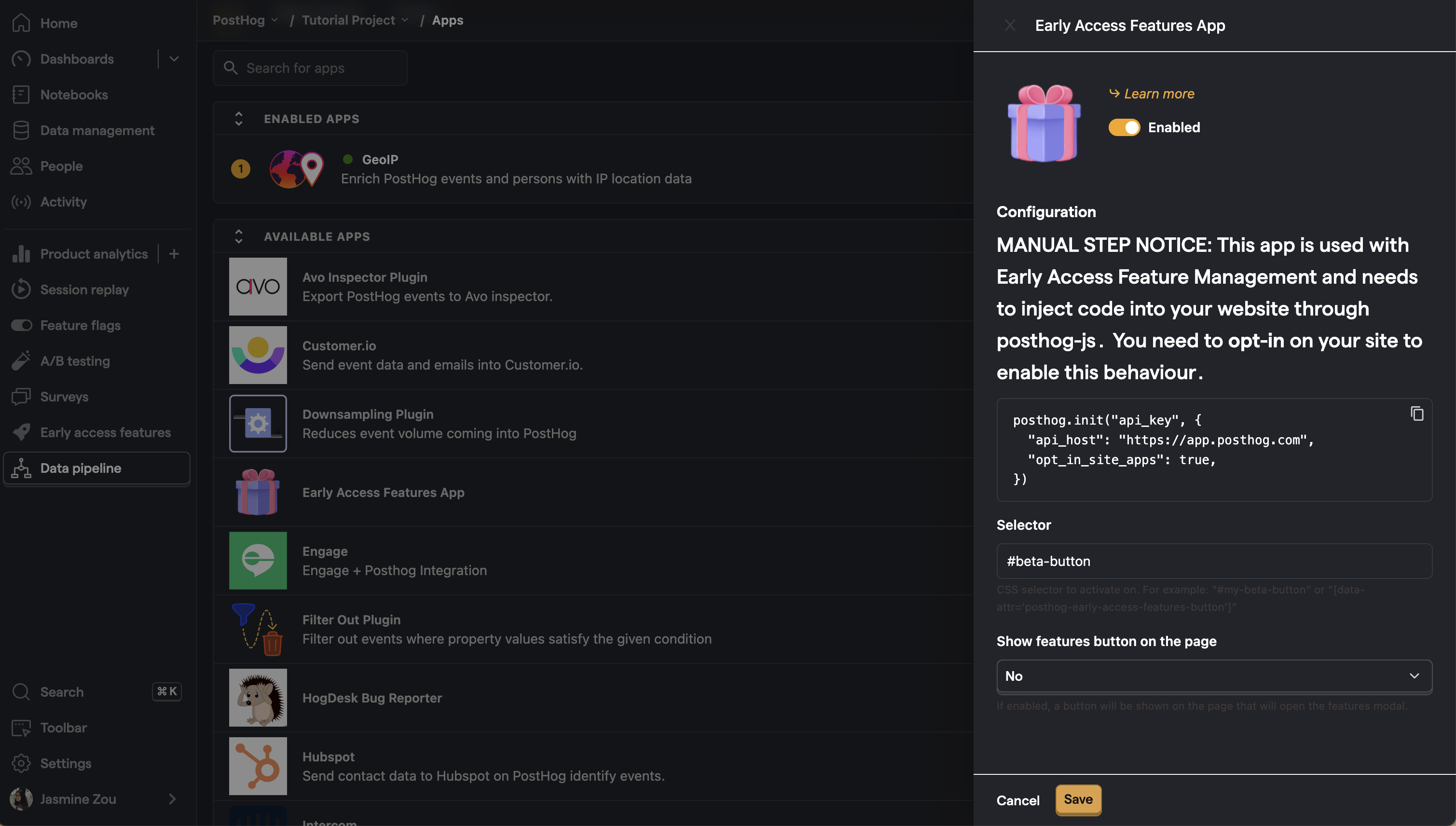
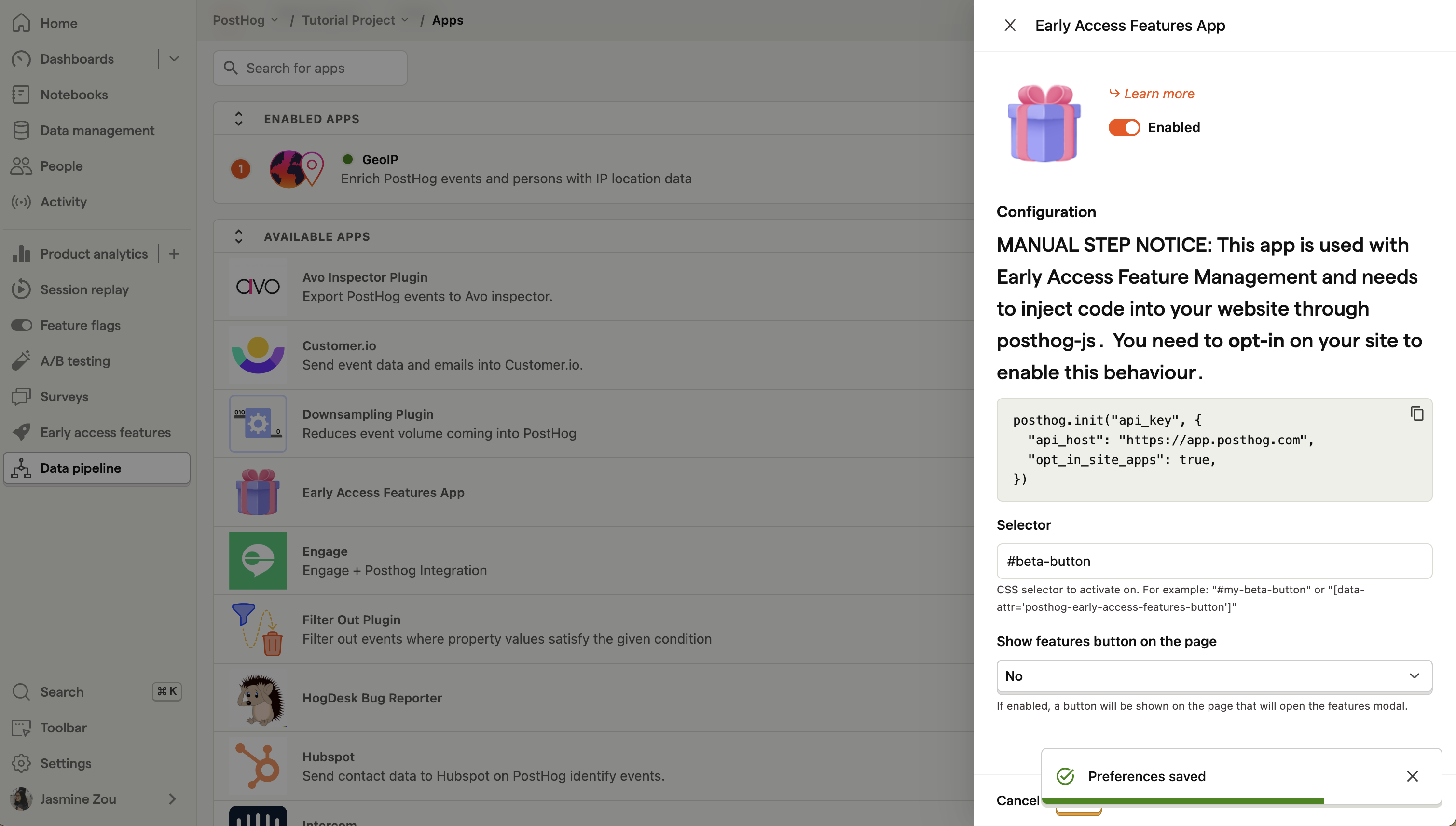
- If you did set an HTML attribute selector, add a component with that selector into your app. For example, if you set your selector to
#beta-button
, you need to add an element like<button id="beta-button">Public Betas</button>
.
Once set up, early access features appear on this panel for users to opt in or out of.
Option 2: Custom implementation
You can fully customize your early access management experience using getEarlyAccessFeatures
and updateEarlyAccessFeatureEnrollment
from PostHog's JavaScript Web library.
getEarlyAccessFeatures
is called with a callback function that receives a list of early access features with their flag key, name, description, and documentation link. You can use this to build a UI for users to opt in or out of features.
Note: Available early access features are cached per browser load. This means if you have a browser open and create a new early access feature, you wouldn't see it until you refresh. If you want the latest values always available, include the
force_reload
parameter in yourgetEarlyAccessFeatures
call. This makes a network request to get the updated list instead of using the cache. The downside is that this can delay when early access features are available on load.
const posthog = usePostHog()const activeFlags = useActiveFeatureFlags()const [activeBetas, setActiveBetas] = useState([])const [inactiveBetas, setInactiveBetas] = useState([])useEffect(() => {posthog.getEarlyAccessFeatures((features) => {if (!activeFlags || activeFlags.length === 0) {setInactiveBetas(features)return}const activeBetas = features.filter(beta => activeFlags.includes(beta.flagKey));const inactiveBetas = features.filter(beta => !activeFlags.includes(beta.flagKey));setActiveBetas(activeBetas)setInactiveBetas(inactiveBetas)}, true)}, [activeFlags])
updateEarlyAccessFeatureEnrollment
is called with a feature flag key and a boolean value to opt in or out of the feature. This updates the user's opt-in status for the feature.
const toggleBeta = (betaKey) => {if (activeBetas.some(beta => beta.flagKey === betaKey)) {posthog.updateEarlyAccessFeatureEnrollment(betaKey,false)setActiveBetas(prevActiveBetas => prevActiveBetas.filter(item => item.flagKey !== betaKey));return}posthog.updateEarlyAccessFeatureEnrollment(betaKey,true)setInactiveBetas(prevInactiveBetas => prevInactiveBetas.filter(item => item.flagKey !== betaKey));}
Other useful functions for custom implementations are isFeatureEnabled()
and useActiveFeatureFlags()
(React only). These help you differentiate users who have opted into early access features from those who have not.
For a sample implementation, see the public beta tutorial here or the site app code here.