Step one: Add PostHog to your app
Our React Native enables you to integrate PostHog with your React Native project. For React Native projects built with Expo, there are no mobile native dependencies outside of supported Expo packages.
To install, add the posthog-react-native
package to your project as well as the required peer dependencies.
Expo apps
npx expo install posthog-react-native expo-file-system expo-application expo-device expo-localization
React Native apps
yarn add posthog-react-native @react-native-async-storage/async-storage react-native-device-info react-native-localize# ornpm i -s posthog-react-native @react-native-async-storage/async-storage react-native-device-info react-native-localize
React Native Web and macOS
If you're using React Native Web or React Native macOS, do not use the expo-file-system package since the Web and macOS targets aren't supported, use the @react-native-async-storage/async-storage package instead.
Configuration
With the PosthogProvider
The recommended way to set up PostHog for React Native is to use the PostHogProvider
. This utilizes the Context API to pass the PostHog client around, enable autocapture, and ensure that the queue is flushed at the right time.
To set up PostHogProvider
, add it to your App.js
or App.ts
file:
// App.(js|ts)import { usePostHog, PostHogProvider } from 'posthog-react-native'...export function MyApp() {return (<PostHogProvider apiKey="<ph_project_api_key>" options={{// usually 'https://us.i.posthog.com' or 'https://eu.i.posthog.com'host: 'https://us.i.posthog.com',}}><MyComponent /></PostHogProvider>)}
Then you can access PostHog using the usePostHog()
hook:
const MyComponent = () => {const posthog = usePostHog()useEffect(() => {posthog.capture("event_name")}, [posthog])}
Without the PosthogProvider
If you prefer not to use the provider, you can initialize PostHog in its own file and import the instance from there:
import PostHog from 'posthog-react-native'export const posthog = new PostHog('<ph_project_api_key>', {// usually 'https://us.i.posthog.com' or 'https://eu.i.posthog.com'host: 'https://us.i.posthog.com'})
Then you can access PostHog by importing your instance:
import { posthog } from './posthog'export function MyApp1() {useEffect(async () => {posthog.capture('event_name')}, [posthog])return <View>Your app code</View>}
You can even use this instance with the PostHogProvider:
import { posthog } from './posthog'export function MyApp() {return <PostHogProvider client={posthog}>{/* Your app code */}</PostHogProvider>}
Requires PostHog React Native SDK version >= 3.2.0, and it's recommended to always use the latest version.
Install the session replay plugin.
yarn add posthog-react-native-session-replay# or npmnpm i -s posthog-react-native-session-replay
Step two: Enable session recordings in your project settings
Enable session recordings in your PostHog Project Settings.
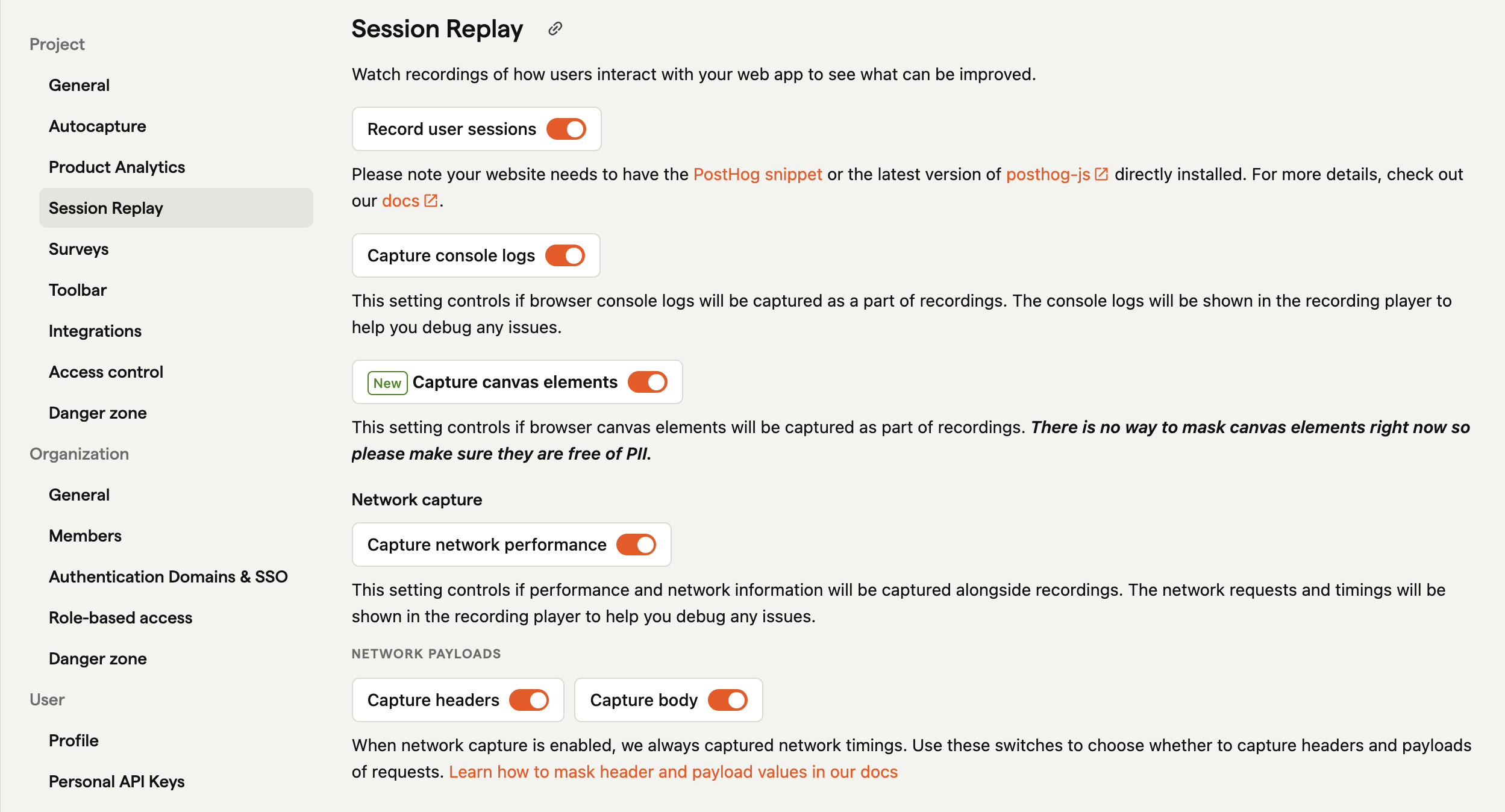
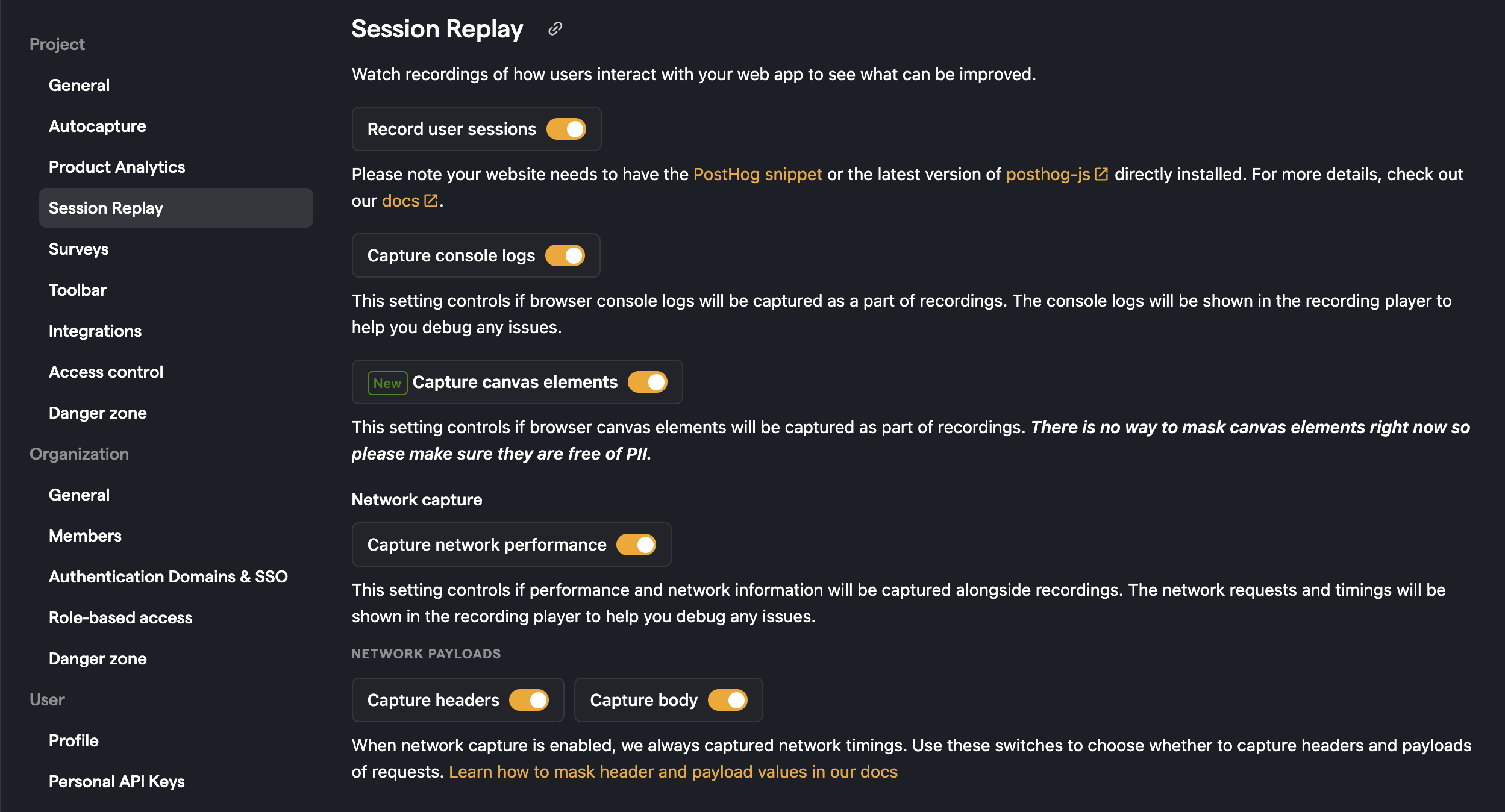
Step three: Configure replay settings
Add enableSessionReplay: true
to your PostHog configuration alongside any of your other configuration options:
export const posthog = new PostHog('<ph_project_api_key>',{// Enable session recording. Requires enabling in your project settings as well.// Default is false.enableSessionReplay: true,sessionReplayConfig: {// Whether text and text input fields are masked. Default is true.// Password inputs are always masked regardlessmaskAllTextInputs: true,// Whether images are masked. Default is true.maskAllImages: true,// Enable masking of all sandboxed system views like UIImagePickerController, PHPickerViewController and CNContactPickerViewController. Default is true.// iOS onlymaskAllSandboxedViews: true,// Capture logs automatically. Default is true.// Android only (Native Logcat only)captureLog: true,// Whether network requests are captured in recordings. Default is true// Only metric-like data like speed, size, and response code are captured.// No data is captured from the request or response body.// iOS onlycaptureNetworkTelemetry: true,// Deboucer delay used to reduce the number of snapshots captured and reduce performance impact. Default is 1000ms// Ps: it was 500ms (0.5s) by default until version 3.3.7androidDebouncerDelayMs: 1000,// Deboucer delay used to reduce the number of snapshots captured and reduce performance impact. Default is 1000msiOSdebouncerDelayMs: 1000,},},);
Or using the PostHogProvider
:
<PostHogProviderapiKey="<ph_project_api_key>"options={{enableSessionReplay: true,...}}>
Expo
Mobile Session Replay for Expo works normally, but prefer the expo
tools for installing and running your project.
Install the session replay plugin.
npx expo install posthog-react-native-session-replay
Expo Go isn't supported since it uses Native plugins, please use development build.
# Run Android development buildnpx expo run:android --no-build-cache --device# Run iOS development buildnpx expo run:ios --no-build-cache --device
Limitations
- On Android, requires API >= 26.
- On iOS, minimum deployment target is iOS13
- Keyboard is not supported. A placeholder will be shown.
- Wireframe mode isn't supported, only screenshot mode.
- Expo Go isn't supported since it uses Native plugins, please use development build.
- Masking individual view components within a WebView isn't supported, if your WebView displays sensitive view components, you've to mask the whole WebView.
Troubleshooting
- Run a clean build if you experience issues such as
The package 'posthog-react-native-session-replay' doesn't seem to be linked
. - Update your SDK and iOS Pods.
- If you have enabled session replay using feature flags, the flags are evaluated on the device once the PostHog SDK starts as early as possible. The SDK might be using the cached flags from the previous SDK start. If you have changed the flag or its condition, kill and reopen the app to force a new SDK start at least once.
- This will also happen in production, and you might experience some delay for the new flag/conditions to take effect on your users. We're tracking this bug here.
- Session replay feature flag evaluation does not capture
$feature_flag_called
events, so theUsage
tab on the feature flag page won't show anything. We're tracking this feature request here.