How to set up Next.js error monitoring
Mar 18, 2025
Errors are an inevitable part of software development, but so is catching and fixing them. You can use error tracking in PostHog to help you do this.
To help you set this up, this tutorial details how to create a basic Next.js app, set up PostHog on both the front and backend, and then automatically capture errors that happen in both locations.
1. Creating a Next.js app
Start by ensuring Node.js is installed (version 18.0 or newer) then run the following command. Say no to TypeScript, yes to app router, and the defaults for other options.
npx create-next-app@latest next-errors
Next, we can create our frontend which will have two parts:
- A button that throws an error
- A button that makes a request to our backend API
We can modify app/page.js
to do this:
'use client'import styles from "./page.module.css";export default function Home() {const handleErrorButtonClick = () => {throw new Error("Frontend error");}const handleAPIButtonClick = async () => {const response = await fetch("/api/test-error");const data = await response.json();console.log("data", data);}return (<div className={styles.page}><h1>Welcome to our broken app</h1><button onClick={handleErrorButtonClick}>Click me for an error</button><button onClick={handleAPIButtonClick}>Click me for a backend API error</button></div>);}
Next, we need to set up our API. To do this, create a new api
directory inside the app
directory, a test-error
directory inside that, and then a route.js
file inside that. In this file, create a basic GET()
function that throws an error like this:
// app/api/test-error/route.jsexport async function GET() {throw new Error('Backend API error')}
Once saved, run npm run dev
to see your new app in action. Click either of the buttons to see the errors they trigger.
2. Setting up PostHog
To start, in your PostHog project settings under error tracking, toggle on Enable exception autocapture. Once done, go back to your app and install both posthog-js
and posthog-node
:
npm i posthog-js posthog-node
Frontend setup
We'll set up PostHog in the frontend first. This starts by creating a providers.js
file in the app
directory. In it, we initialize PostHog with your project API key and host from your project settings and pass it to a PostHogProvider
.
// app/providers.js'use client'import posthog from 'posthog-js'import { PostHogProvider as PHProvider } from 'posthog-js/react'import { useEffect } from 'react'export function PostHogProvider({ children }) {useEffect(() => {posthog.init('<ph_project_api_key>', {api_host: 'https://us.i.posthog.com',defaults: '2025-05-24',})}, [])return (<PHProvider client={posthog}>{children}</PHProvider>)}
We then import this into layout.js
and wrap our app in it like this:
import "./globals.css";import { PostHogProvider } from "./providers";export default function RootLayout({ children }) {return (<html lang="en"><body><PostHogProvider>{children}</PostHogProvider></body></html>);}
PostHog then begins to autocapture events and frontend errors. If you go back to your app and click the Click me for an error button, you'll see an $exception
event captured into PostHog.
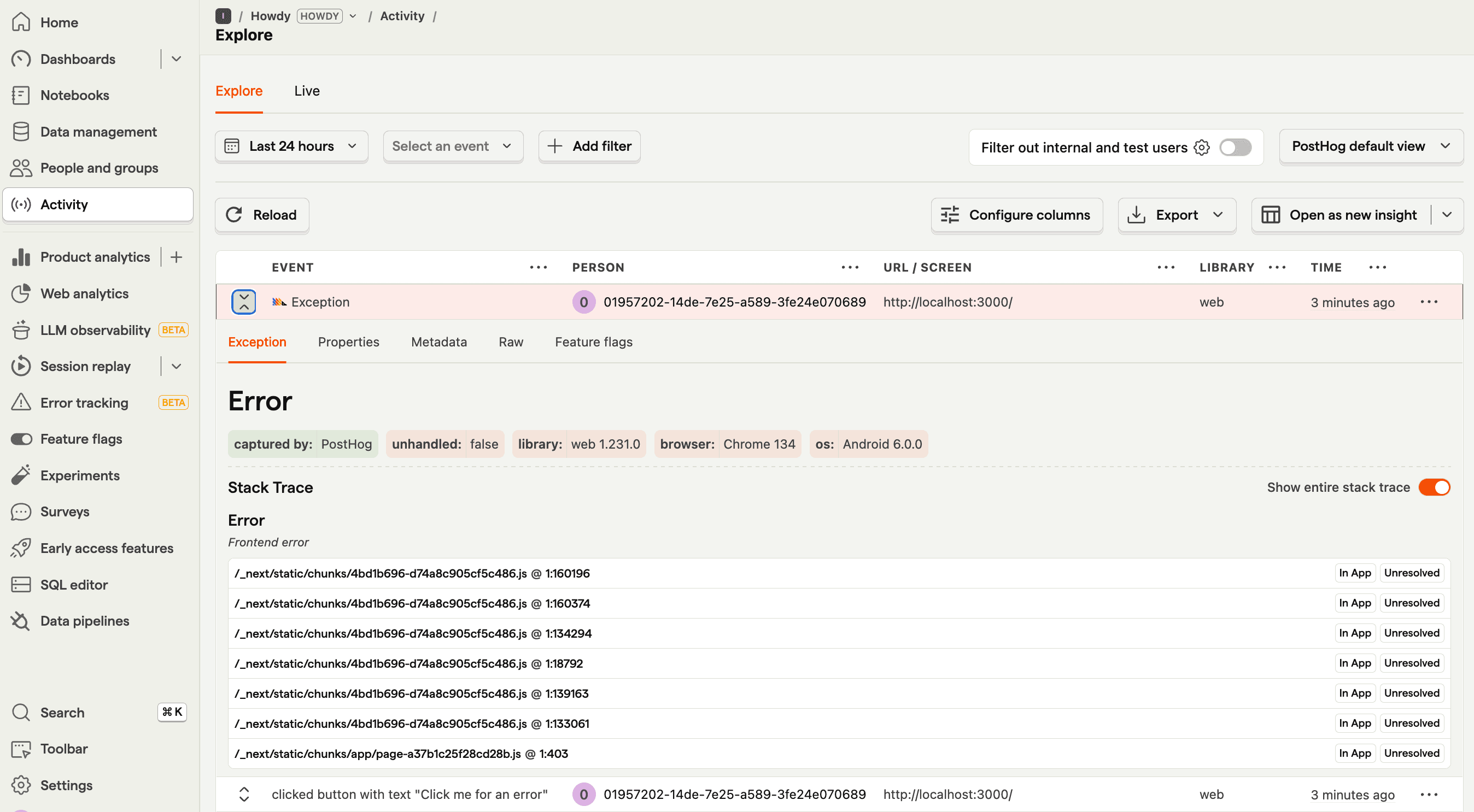
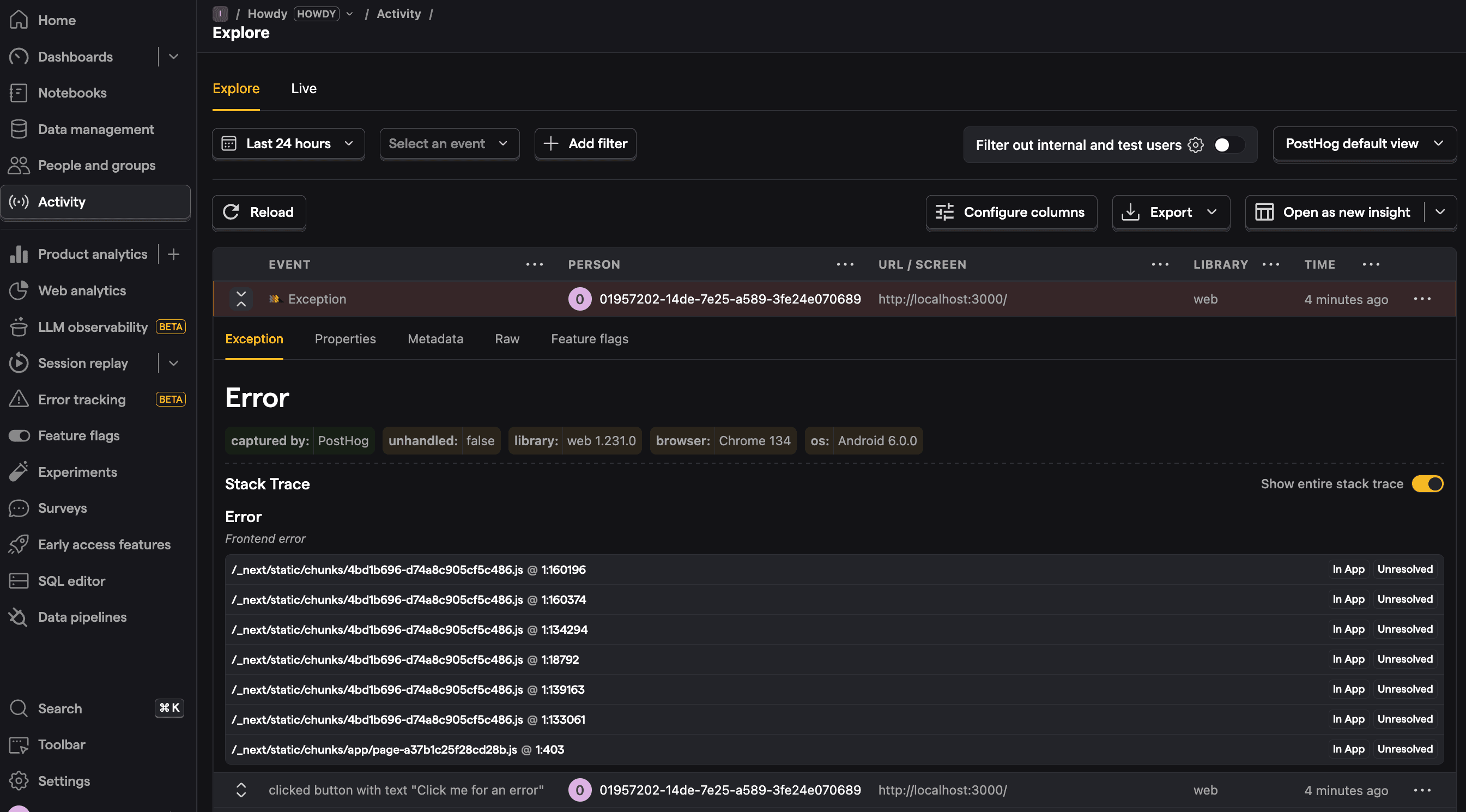
Backend setup
For the backend, we can create a posthog-server.js
file in the app
directory. In it, initialize PostHog from posthog-node
as a singleton with your project API key and host from your project settings. This looks like this:
// app/posthog-server.jsimport { PostHog } from 'posthog-node'let posthogInstance = nullexport function getPostHogServer() {if (!posthogInstance) {posthogInstance = new PostHog('<ph_project_api_key>',{host: 'https://us.i.posthog.com',flushAt: 1,flushInterval: 0})}return posthogInstance}
We can then import this singleton wherever we need it in the backend. Unfortunately, this doesn't autocapture errors by default, so we have some more work to do.
3. Capturing errors
With both front and backend initializations set up, capturing errors with PostHog is as simple as calling captureException
or capturing an $exception
event.
posthog.captureException(error, additionalProperties)
Doing this for every possible error is a hassle though and we'll inevitably miss errors we're not expecting. Our frontend implementation automatically captures errors thrown and caught by onError
and onUnhandledRejection
listeners, but this doesn't cover everything.
To capture more, we can set up some more boundaries and instrumentation.
How to capture frontend render errors
To ensure all component errors are tracked, we can use the built-in error boundary system. This is done by creating an error.jsx
file like this:
// app/error.jsx'use client'import { useEffect } from 'react'import posthog from 'posthog-js'export default function Error({error,reset,}) {useEffect(() => {posthog.captureException(error)}, [error])return (<div><h1>Something went wrong!</h1><p>We've logged this error and will look into it.</p><button onClick={() => reset()}>Try again</button></div>)}
This triggers when there is an error rendering your component. You can test this by setting up a useEffect
in our page.js
file that triggers a render error like this:
'use client'import { useState, useEffect } from 'react'// ... rest of your codeconst [shouldError, setShouldError] = useState(false)useEffect(() => {setShouldError(true)}, [])if (shouldError) {throw new Error('This is a test error')}// ... rest of your code
You can also create a similar global-error.jsx
file to capture errors affecting the root layout or more granular error boundaries by adding error.jsx
files to specific route segments.
How to automatically capture backend errors
Because backend requests in Next.js vary between server-side rendering, short-lived processes and more, we can't rely on exception autocapture.
Instead, we create a instrumentation.js
file at the root of our project and set up an onRequestError
handler there. Importantly, we to both check the request is running in the nodejs
runtime to ensure PostHog works and get the distinct_id
from the cookie to connect the error to a specific user.
This looks like this:
// instrumentation.jsexport function register() {// No-op for initialization}export const onRequestError = async (err, request, context) => {if (process.env.NEXT_RUNTIME === 'nodejs') {const { getPostHogServer } = require('./app/posthog-server')const posthog = await getPostHogServer()let distinctId = nullif (request.headers.cookie) {const cookieString = request.headers.cookieconst postHogCookieMatch = cookieString.match(/ph_phc_.*?_posthog=([^;]+)/)if (postHogCookieMatch && postHogCookieMatch[1]) {try {const decodedCookie = decodeURIComponent(postHogCookieMatch[1])const postHogData = JSON.parse(decodedCookie)distinctId = postHogData.distinct_id} catch (e) {console.error('Error parsing PostHog cookie:', e)}}}await posthog.captureException(err, distinctId || undefined)}}
Now, when you click the Click me for a backend API error button, it will trigger an error which will be automatically captured by PostHog.
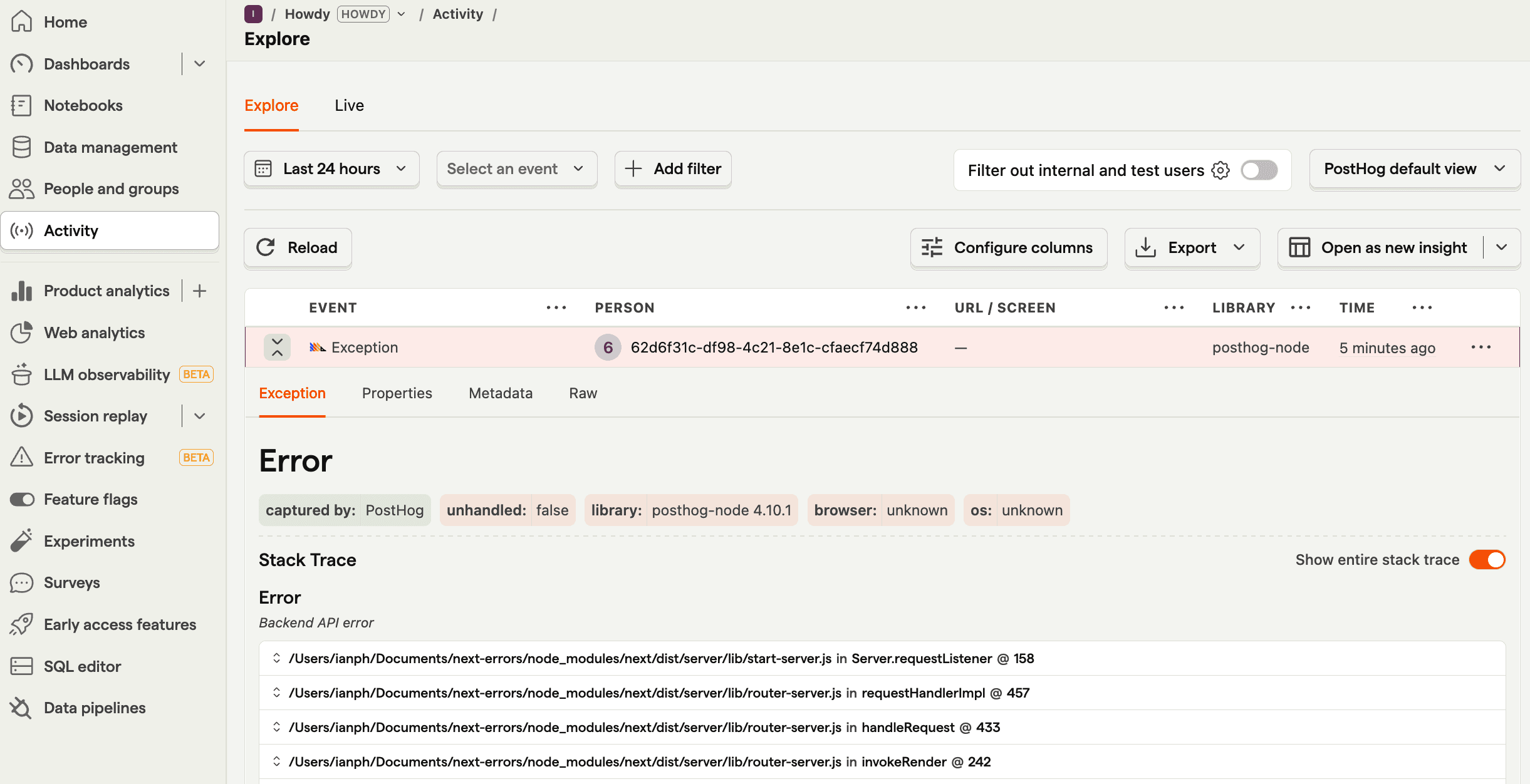
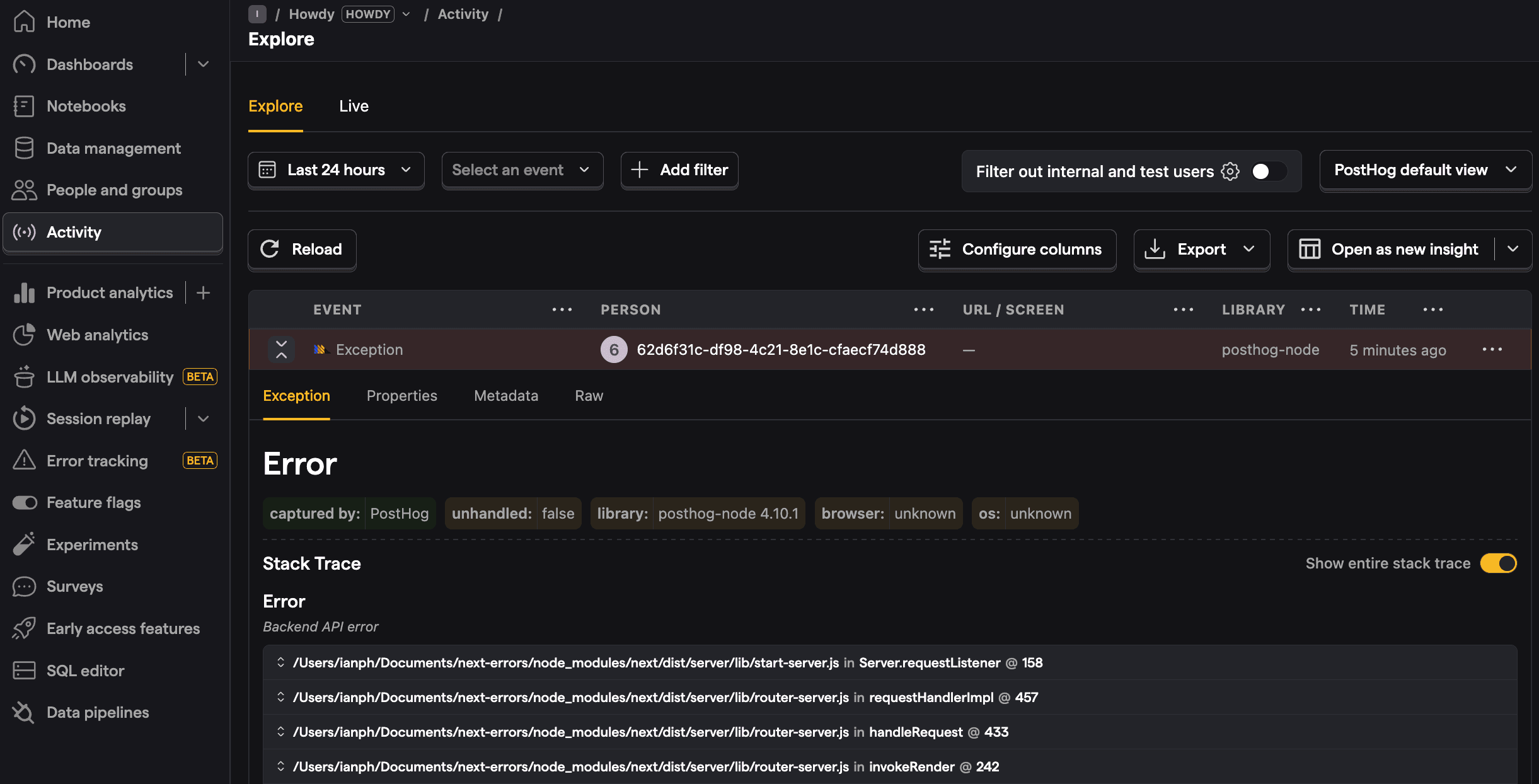
4. Monitoring errors in PostHog
Once you've set up error capture in your app, you can head to the error tracking tab in PostHog to review the issues popping up along with their frequency.
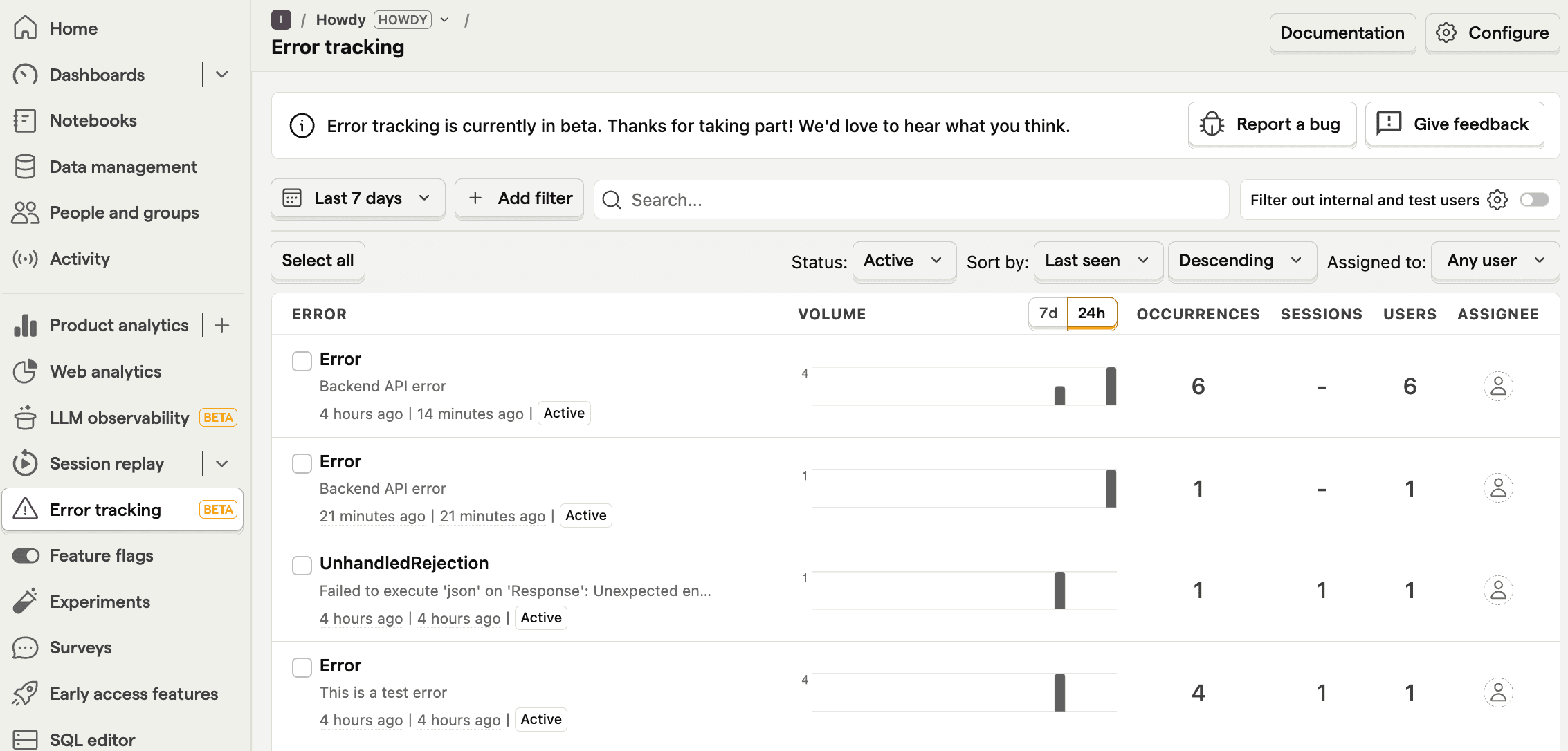
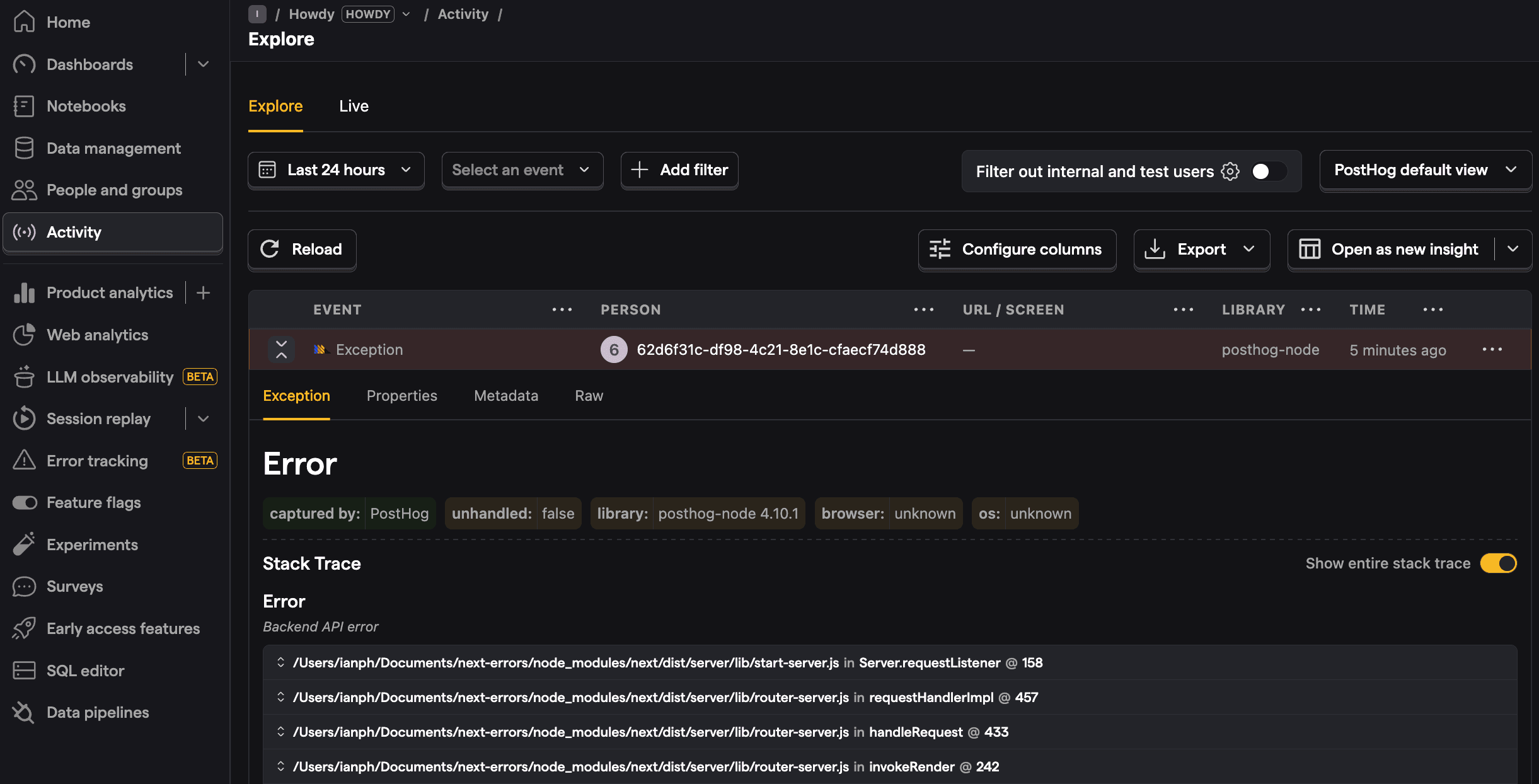
You can click into any of these errors to get more details on them, including a stack trace as well as archive, resolve, or suppress them. On top of this, you can analyze $exception
events like you would any event in PostHog, including setting up trends for them and querying them with SQL.
5. Uploading source maps
We provide a helper package that will hook into the Next.js build process and upload sourcemaps for your client and server code. This process is enabled by default for production builds but you can disable it by setting enabled
to false
in the sourcemaps
object.
1. Install package
npm install @posthog/nextjs-config
2. Update your next config
import { withPostHogConfig } from "@posthog/nextjs-config";const nextConfig = {//...your nextjs config,};export default withPostHogConfig(nextConfig, {personalApiKey: process.env.POSTHOG_API_KEY, // Personal API KeyenvId: process.env.POSTHOG_ENV_ID, // Environment IDhost: process.env.NEXT_PUBLIC_POSTHOG_HOST, // (optional), defaults to https://us.posthog.comsourcemaps: { // (optional)enabled: true, // (optional) Enable sourcemaps generation and upload, default to true on production buildsproject: "my-application", // (optional) Project name, defaults to repository nameversion: "1.0.0", // (optional) Release version, defaults to current git commitdeleteAfterUpload: true, // (optional) Delete sourcemaps after upload, defaults to true},});
Where you should set the following environment variables:
Environment Variable | Description |
---|---|
POSTHOG_API_KEY | Personal API key with at least write access on error tracking |
POSTHOG_ENV_ID | Project ID you can find in your project settings |
NEXT_PUBLIC_POSTHOG_HOST | Your PostHog instance URL. Defaults to https://us.posthog.com |
That's it!
Now when you build your application with next build
, your sourcemaps are uploaded to PostHog and you will be able to see the unminified code your error originated from in your dashboard. This step is disabled by default with next dev
.
Questions? Ask Max AI.
It's easier than reading through 680 pages of documentation